forked from github/paste-markdown
-
Notifications
You must be signed in to change notification settings - Fork 0
/
Copy pathtest.js
130 lines (117 loc) · 4 KB
/
test.js
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
import subscribe from '../dist/index.esm.js'
describe('paste-markdown', function () {
describe('installed on textarea', function () {
let subscription, textarea
beforeEach(function () {
document.body.innerHTML = `
<textarea data-paste-markdown></textarea>
`
textarea = document.querySelector('textarea[data-paste-markdown]')
subscription = subscribe(textarea)
})
afterEach(function () {
subscription.unsubscribe()
document.body.innerHTML = ''
})
it('turns image uris into markdown', function () {
paste(textarea, {'text/uri-list': 'https://github.com/github.png\r\nhttps://github.com/hubot.png'})
assert.include(textarea.value, '\n\n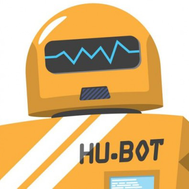')
})
it('turns html tables into markdown', function () {
const data = {
'text/html': `
<table>
<thead><tr><th>name</th><th>origin</th></tr></thead>
<tbody>
<tr><td>hubot</td><td>github</td></tr>
<tr><td>bender</td><td>futurama</td></tr>
</tbody>
</table>
`
}
paste(textarea, data)
assert.include(textarea.value, 'name | origin\n-- | --\nhubot | github\nbender | futurama')
})
it('retains text around tables', async function () {
const data = {
'text/html': `
<p>Here is a cool table</p>
<table>
<thead><tr><th>name</th><th>origin</th></tr></thead>
<tbody>
<tr><td>hubot</td><td>github</td></tr>
<tr><td>bender</td><td>futurama</td></tr>
</tbody>
</table>
<p>Very cool</p>
`
}
paste(textarea, data)
assert.equal(
textarea.value.trim(),
// eslint-disable-next-line github/unescaped-html-literal
'<p>Here is a cool table</p>\n \nname | origin\n-- | --\nhubot | github\nbender | futurama\n\n\n <p>Very cool</p>'
)
})
it('pastes multiple tables', async function () {
const data = {
'text/html': `
<p>Here is a cool table</p>
<table>
<thead><tr><th>name</th><th>origin</th></tr></thead>
<tbody>
<tr><td>hubot</td><td>github</td></tr>
<tr><td>bender</td><td>futurama</td></tr>
</tbody>
</table>
<p>Another very cool table</p>
<table>
<thead><tr><th>name</th><th>origin</th></tr></thead>
<tbody>
<tr><td>hubot</td><td>github</td></tr>
<tr><td>bender</td><td>futurama</td></tr>
</tbody>
</table>
`
}
paste(textarea, data)
assert.equal(
textarea.value.trim(),
// eslint-disable-next-line github/unescaped-html-literal
'<p>Here is a cool table</p>\n \nname | origin\n-- | --\nhubot | github\nbender | futurama\n\n\n <p>Another very cool table</p>\n \nname | origin\n-- | --\nhubot | github\nbender | futurama'
)
})
it('rejects layout tables', function () {
const data = {
'text/html': `
<table data-paste-markdown-skip>
<thead><tr><th>name</th><th>origin</th></tr></thead>
<tbody>
<tr><td>hubot</td><td>github</td></tr>
<tr><td>bender</td><td>futurama</td></tr>
</tbody>
</table>
`
}
paste(textarea, data)
assert.equal(
textarea.value,
'\n \n nameorigin\n \n hubotgithub\n benderfuturama\n \n \n '
)
})
it('accepts x-gfm', function () {
paste(textarea, {'text/plain': 'hello', 'text/x-gfm': '# hello'})
assert.include(textarea.value, '# hello')
})
})
})
function paste(textarea, data) {
const dataTransfer = new DataTransfer()
for (const key in data) {
dataTransfer.setData(key, data[key])
}
const event = new ClipboardEvent('paste', {
clipboardData: dataTransfer
})
textarea.dispatchEvent(event)
}