-
Notifications
You must be signed in to change notification settings - Fork 1
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Possible buggy heuristic implementation #1
Comments
Hi @dingyuchen! Thanks for checking out my project. You are correct that the algorithm isn't implemented exactly the same as paper on the line in question (although they are equivalent). I went back and reviewed the paper and algorithm to see how I arrived at my implementation.
The algorithm, as you have posted above, reads as follows (for each row, summarized):
Our board:
Now let's walk through the algorithm on the row of interest Step 1:
Final conflict counts: [2, 2, 2] Step 2:
So I arrive at a total count of 4. However, there is more to the full implementation that I provide here (and as described in the paper).
Our final total should be 8. I have also validated this algortihm against BFS on all possible 3x3, 3x4, 4x3, and 4x4 boards. Although that isn't a proof, it does increase my confidence that I am at least not overcounting, as we would have almost certainly hit at least 1 board where the solution overshoots the distance and does not match BFS. (And in fact, in earlier versions of my code there were bugs that were caught by running through all possible boards.) |
Re-opening b/c exhaustive test is now failing after latest change:
|
I see, I realised I missed out on the initialization value of
However, I still have doubt that this is equivalent to the algorithm published in the paper. If I have a 15puzzle with first row [2, 1, 4, 3]. My final conflict count would be [1, 1, 1, 1]. |
You are correct that I should not decrement all other conflicts---only the tiles that we were originally in conflict with. I recall this is the reason I originally implemented it by setting the largest conflicting tile to be a "fake" blank, and then simply recompute conflicts from scratch. I found this to be easier to reason about. I'm going to change that back. Secondly, the error above is actually unrelated to my recent change---it is due to last moves/corner tiles distance. When that is removed, the exhaustive search actually passes. It appears my |
Okay, figured it all out. Multiple bugs here:
On this board, the 8 tile move distance is already accounted for by bottom-left corner heuristic, but then is double-counted by the last moves heuristic. An analogous situation happens at top of board:
Here, the top-left and top-right corners double-count the 4, b/c there is no gap between corner-adjacent tiles on such small boards. I have also added:
|
slidingpuzzle/src/slidingpuzzle/heuristics.py
Line 328 in 458308d
According to _hannson, the conflict count for a row should be the number of tiles needed to be removed to resolve the conflict, times 2. For example, for a the middle row in an 8-puzzle, [6, 5, 4] should have 2 * 2 added to the heuristic value; 6 and 5 can be moved out of the row, 4 slides into position while staying on the row, 5 and 6 slides back.
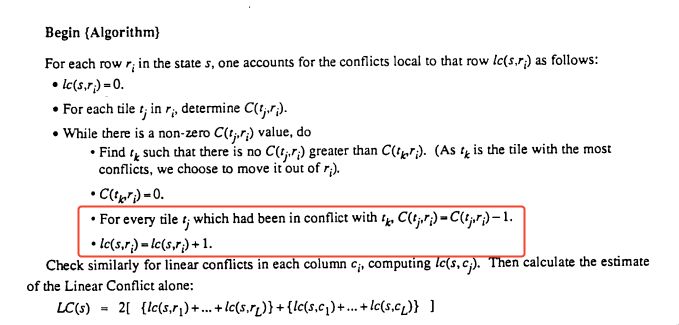
Cmiiw, your implementation does not account for this detail.
slidingpuzzle/tests/test_heuristics.py
Line 87 in 458308d
I believe the testcase should also be 6 instead of 8
The text was updated successfully, but these errors were encountered: