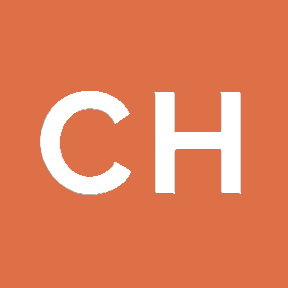
Application Serverless ETL Query and Resource API
for @israelias/cheathub/frontend
Explore the docs »
View API
·
Report Bug
·
Create a PR
This is the backend of a monorepo. It serves API routes, controls API methods via a simple pattern of class-resources-database-routes
a la Flask app with an opinionated directory set up (#wantstobedjango).
- Models extend from
db.Document
viadb = MongoEngine()
- Resource classes extend from
flask_restful.Resource
- Routes are served via
flask_restful.API
This repository separates concerns and avoids circular imports by creating init
functions for each module ({module}.init_app(app)
) so that the root app.py
can be as clean as follows:
initialize_db(app)
initialize_routes(api)
initialize_basicauth(app)
initialize_admin(app)
initialize_views()
- The restful API is deployed at cheathub-backend.herokuapp.com/
- MkDocs for the DB/API is deployed at israelias.github.io/chub-etl-api
Note: Open API spec is forthcoming. Please see
database/models.py
to preview the snippet, collection and user document models.
Don't do it this way, but sadly, here we are
It's currently a heroku deployment on the backend. If you so choose to go this route, this is how it happened. The project is currently being moved to a different server because Heroku is not ideal.
#create new git repository and add everything
git init
git add .
git commit -m "Initialize for Heroku deployment"
# heroku git:remote -a cheathub-backend
git remote add heroku [email protected]:cheathub-backend.git
#pull heroku but then checkback out our current local master and mark everything as merged
git pull heroku master
git checkout --ours .
git add -u
git commit -m "merged"
#push back to heroku, open web browser, and remove git repository
git push heroku master
heroku open
rm -fr .git
#go back to wherever we started.
cd -
heroku git:remote -a cheathub-backend
git push heroku master
The latest releases Python 3.4+ and Python 2.7.9+, as well as the virtual environments
virtualenv
andpyvenv
, automatically ship with PIP
- Please have
python3
globally installed on your machine. - Please set environment variables-> jump to
env.py
- Clone the Mono Repo
git clone ssh://[email protected]/israelias/cheathub.git
- Jump into this directory
cd cheathub/backend
- Create a Virtual Env
python -m venv venv
- Activate your environment
source venv/bin/activate
- Install existing
requirements
(venv) pip install -r requirements.txt
- Run the development environment (Assumes
env.py
is set)(venv) python run.py
In this half of the monorepo, you can run:
- Serves the Databse backend
- There is no local/prod separation here, we connect to Mongo and that is that.
- Alternatively, you can connect to a local db by replacing the db instance
- Runs the backend app in the development mode.
- All this means is that debug mode is on
- Open http://localhost:5000/admin to view the admin panel in the browser (ensure
BASIC_AUTH
variables are set). - Open http://localhost:5000/api/snippets to view the JSON response format of all public snippets -- assuming
snippet
objects have been created. Otherwise, use Postman to create documents locally (API spec is forthcoming!).
The page will reload if you make edits. You will also see any errors in the console.
- /docs (Currently served from israelias/chub-etl-api which is a clone of this backend for testing various servers with a reliable Python runtime that is not Heroku)
- /admin
- /api/snippets
To recreate this backend app and database from scratch add the forthcoming environment variables to a local env.py
.
# as written in app.py
app.config['SAMPLE_ENV_VAR'] = os.environ.get("SAMPLE_ENV_VAR")
The code is written in the app's Flask instance to auto-configure with these env.py
variables:
A connection string from Mongo Atlas for Mongo Engine
to connect to remotely.
To recreate:
- Create an account with MongoDB Atlas.
- Create a Cluster named
hub
. - Create a Database named
cheathubdb
. - Click
Connect
to generate a connection string.
Set the variable to this connection string:
# env.py
os.environ.setdefault("MONGODB_HOST", "mongodb+srv://<username>:<password>@hub.4kotr.mongodb.net/cheathubdb?retryWrites=true&w=majority")
Any key for Sessions
to work.
Set the variable to this key:
# env.py
os.environ.setdefault("SECRET_KEY", "<your secret key>")
The port to connect to.
Set the variable to this key:
# env.py
os.environ.setdefault("MONGODB_PORT", "5000")
Any string key for JWT to work.
Set the variable to this key:
# env.py
os.environ.setdefault("JWT_SECRET_KEY", "<your JWT secret key>")
Superuser username to access admin panel at /admin
Set the variable to this key:
# env.py
os.environ.setdefault("BASIC_AUTH_USERNAME", "<your superuser username>")
Superuser password to access admin panel at /admin
Set the variable to this key:
# env.py
os.environ.setdefault("BASIC_AUTH_USERNAME", "<your superuser password>")
See Flask Mail Similarly, apply the same logic to:
MAIL_SERVER
MAIL_PORT
MAIL_USERNAME
MAIL_PASSWORD
MAIL_DEFAULT_SENDER
The Collections named snippet
, user
, and collection
will automatically be created when documents are made following the classes in this repo. You can also create these collections manually, and add documents on the atlas Cloud interface or Mongo Compass.
You can interact with the database via Mongo shell:
show dbs
use cheathubdb
show collections
db.users.find().pretty()
You can add documents via Postman or Mongo Compass.