|
| 1 | +这篇文章,我就简单给大家聊聊项目必备的 Swagger 该怎么玩。 |
| 2 | + |
| 3 | +**何为 Swagger ?** 简单来说,Swagger 就是一套基于 OpenAPI 规范构建的开源工具,可以帮助我们设计、构建、记录以及使用 Rest API。 |
| 4 | + |
| 5 | +**为何要用 Swagger ?** 前后端分离的情况下,一份 Rest API 文档将会极大的提高我们的工作效率。前端小伙伴只需要对照着 Rest API 文档就可以搞清楚一个接口需要的参数以及返回值。通过 Swagger 我们只需要少量注解即可生成一份自带 UI 界面的 Rest API 文档,不需要我们后端手动编写。并且,通过 UI 界面,我们还可以直接对相应的 API 进行调试,省去了准备复杂的调用参数的过程。 |
| 6 | + |
| 7 | +这篇文章的主要内容: |
| 8 | + |
| 9 | +1. SpringBoot 项目中如何使用? |
| 10 | +2. Spring Security 项目中如何使用? |
| 11 | +3. 使用 knife4j 增强 Swagger |
| 12 | + |
| 13 | +以下演示所用代码,你可以在这个仓库找到:[https://github.com/Snailclimb/spring-security-jwt-guide](https://github.com/Snailclimb/spring-security-jwt-guide) (从零入门 !Spring Security With JWT(含权限验证)后端部分代码) |
| 14 | + |
| 15 | +## SpringBoot 项目中如何使用? |
| 16 | + |
| 17 | +Swagger3.0 官方已经有了自己的 Spring Boot Starter,只需要添加一个 jar 包即可(SpringBoot 版本 2.3.6.RELEASE)。。 |
| 18 | + |
| 19 | +```xml |
| 20 | +<!-- swagger --> |
| 21 | +<dependency> |
| 22 | + <groupId>io.springfox</groupId> |
| 23 | + <artifactId>springfox-boot-starter</artifactId> |
| 24 | + <version>3.0.0</version> |
| 25 | +</dependency> |
| 26 | +``` |
| 27 | + |
| 28 | +什么都不用配置!直接在浏览器中访问 :[http://ip:port/swagger-ui/](http://ip:port/swagger-ui/) 即可。 |
| 29 | + |
| 30 | +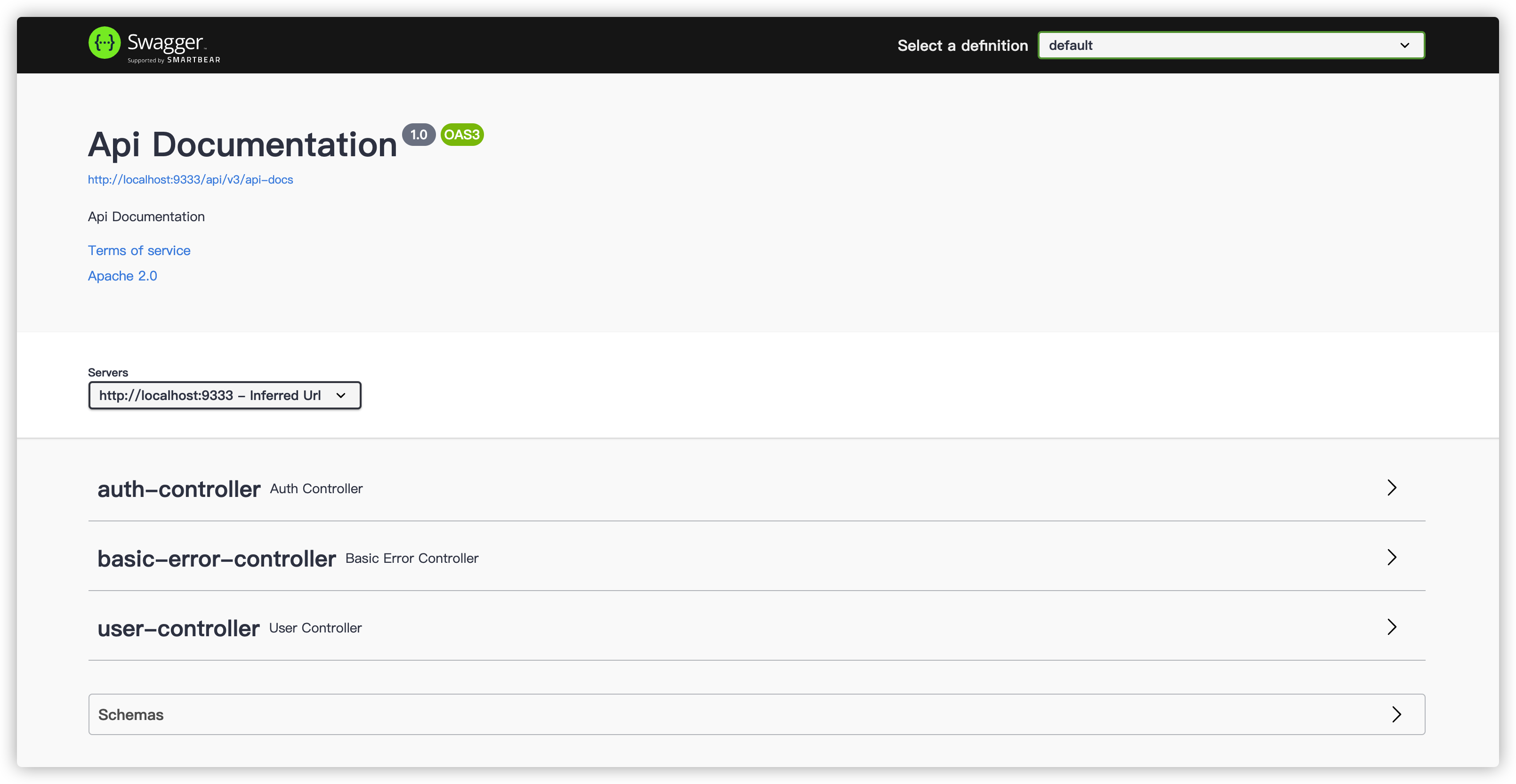 |
| 31 | + |
| 32 | +## Spring Security 项目中如何使用? |
| 33 | + |
| 34 | +如果你的项目使用了 Spring Security 做权限认证的话,你需要为 Swagger 相关 url 添加白名单。 |
| 35 | + |
| 36 | +```java |
| 37 | + String[] SWAGGER_WHITELIST = { |
| 38 | + "/swagger-ui.html", |
| 39 | + "/swagger-ui/*", |
| 40 | + "/swagger-resources/**", |
| 41 | + "/v2/api-docs", |
| 42 | + "/v3/api-docs", |
| 43 | + "/webjars/**" |
| 44 | + }; |
| 45 | + |
| 46 | + @Override |
| 47 | + protected void configure(HttpSecurity http) throws Exception { |
| 48 | + http.cors().and() |
| 49 | + // 禁用 CSRF |
| 50 | + .csrf().disable() |
| 51 | + .authorizeRequests() |
| 52 | + // swagger |
| 53 | + .antMatchers(SWAGGER_WHITELIST).permitAll() |
| 54 | + ...... |
| 55 | + } |
| 56 | +``` |
| 57 | + |
| 58 | +另外,某些请求需要认证之后才可以访问,为此,我们需要对 Swagger 做一些简单的配置。 |
| 59 | + |
| 60 | +配置的方式非常简单,我提供两种不同的方式给小伙伴们。 |
| 61 | + |
| 62 | +1. 登录后自动为请求添加 token。 |
| 63 | +2. 为请求的 Header 添加一个认证参数,每次请求的时候,我们需要手动输入 token。 |
| 64 | + |
| 65 | +### 登录后自动为请求添加 token |
| 66 | + |
| 67 | +通过这种方式我们只需要授权一次即可使用所有需要授权的接口。 |
| 68 | + |
| 69 | +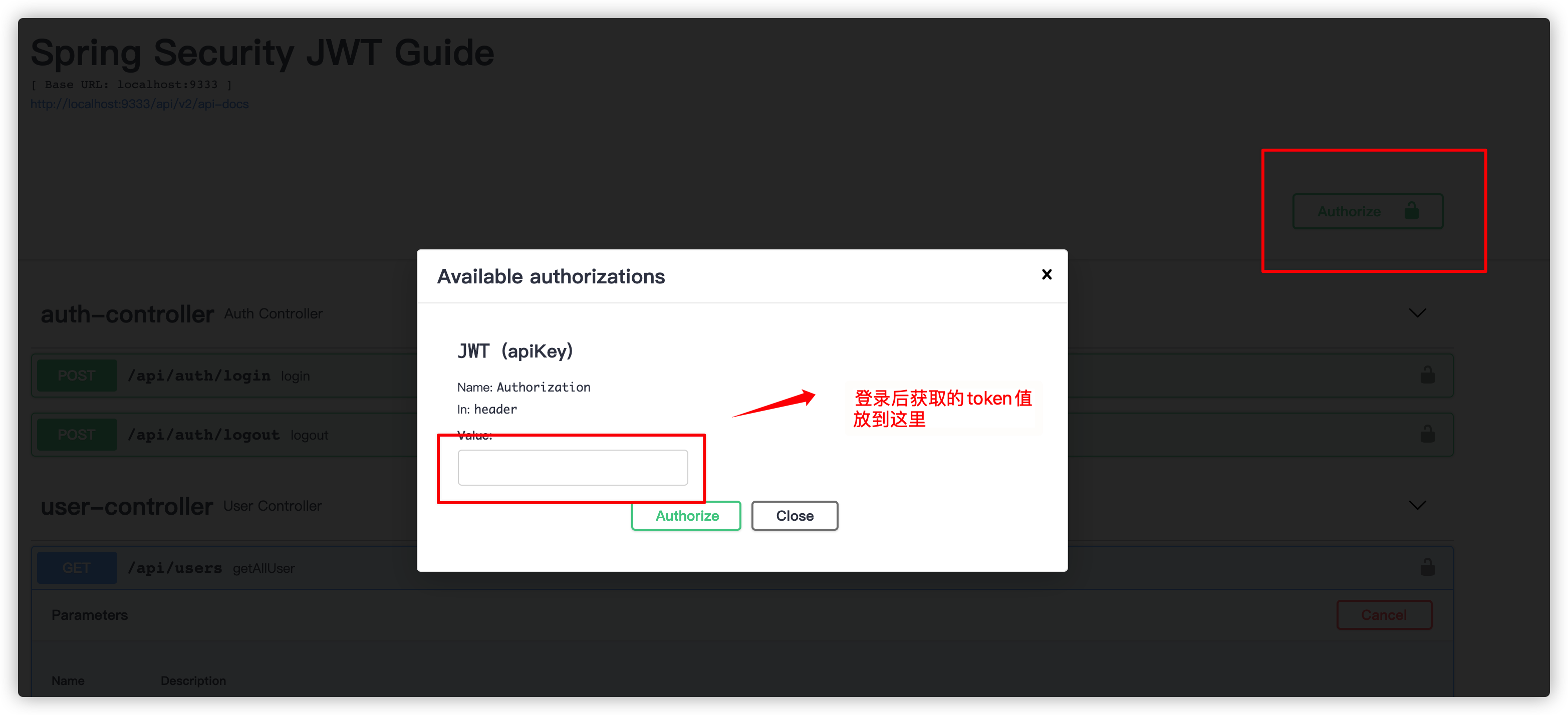 |
| 70 | + |
| 71 | +```java |
| 72 | +/** |
| 73 | + * @author shuang.kou |
| 74 | + * @description swagger 相关配置 |
| 75 | + */ |
| 76 | +@Configuration |
| 77 | +public class SwaggerConfig { |
| 78 | + |
| 79 | + @Bean |
| 80 | + public Docket createRestApi() { |
| 81 | + return new Docket(DocumentationType.SWAGGER_2) |
| 82 | + .apiInfo(apiInfo()) |
| 83 | + .select() |
| 84 | + .apis(RequestHandlerSelectors.basePackage("github.javaguide.springsecurityjwtguide")) |
| 85 | + .paths(PathSelectors.any()) |
| 86 | + .build() |
| 87 | + .securityContexts(securityContext()) |
| 88 | + .securitySchemes(securitySchemes()); |
| 89 | + } |
| 90 | + |
| 91 | + private List<SecurityScheme> securitySchemes() { |
| 92 | + return Collections.singletonList(new ApiKey("JWT", SecurityConstants.TOKEN_HEADER, "header")); |
| 93 | + } |
| 94 | + |
| 95 | + private List<SecurityContext> securityContext() { |
| 96 | + SecurityContext securityContext = SecurityContext.builder() |
| 97 | + .securityReferences(defaultAuth()) |
| 98 | + .build(); |
| 99 | + return Collections.singletonList(securityContext); |
| 100 | + } |
| 101 | + |
| 102 | + List<SecurityReference> defaultAuth() { |
| 103 | + AuthorizationScope authorizationScope |
| 104 | + = new AuthorizationScope("global", "accessEverything"); |
| 105 | + AuthorizationScope[] authorizationScopes = new AuthorizationScope[1]; |
| 106 | + authorizationScopes[0] = authorizationScope; |
| 107 | + return Collections.singletonList(new SecurityReference("JWT", authorizationScopes)); |
| 108 | + } |
| 109 | + |
| 110 | + private ApiInfo apiInfo() { |
| 111 | + return new ApiInfoBuilder() |
| 112 | + .title("Spring Security JWT Guide") |
| 113 | + .build(); |
| 114 | + } |
| 115 | +} |
| 116 | +``` |
| 117 | + |
| 118 | +**未登录前:** |
| 119 | + |
| 120 | +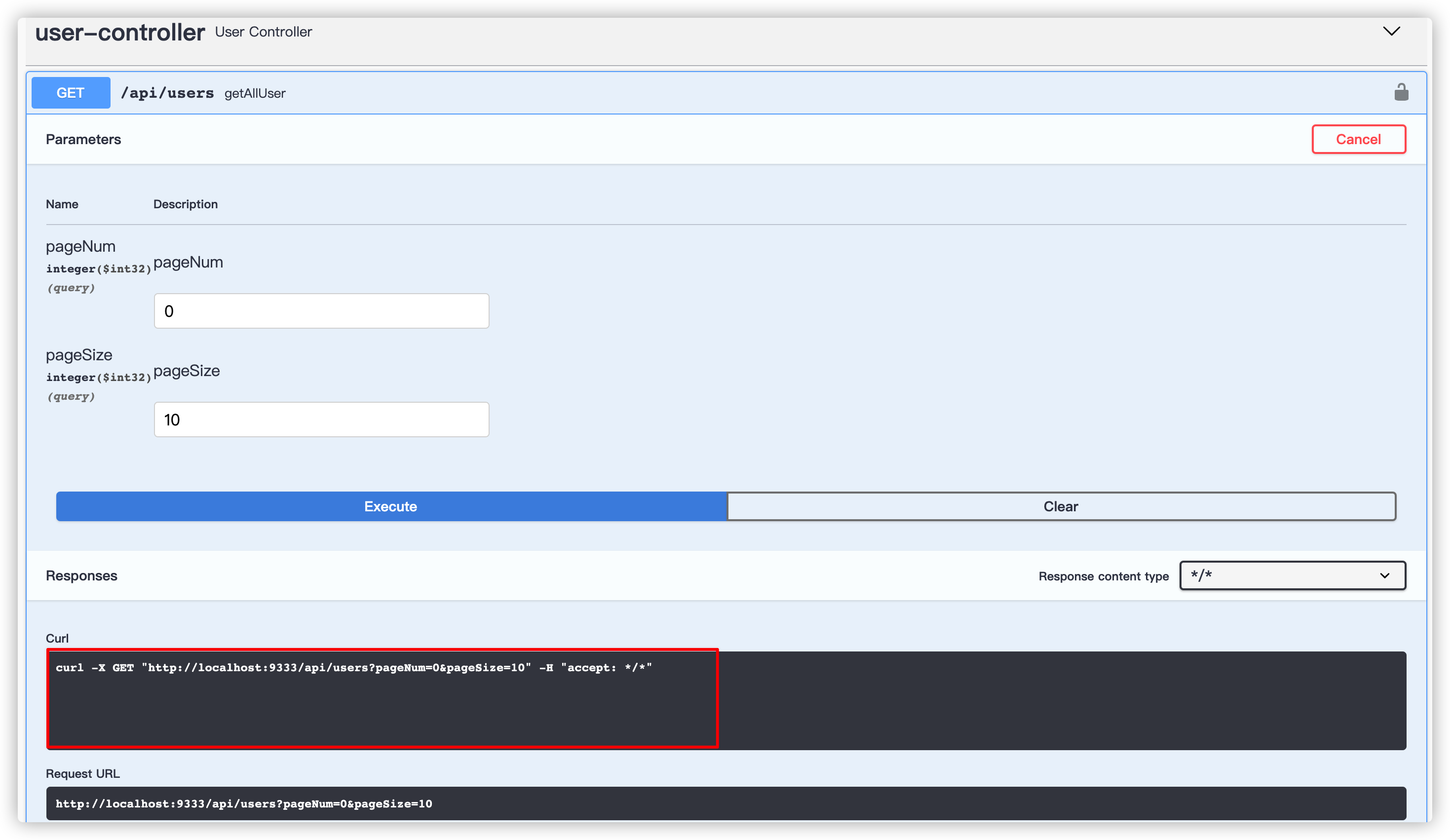 |
| 121 | + |
| 122 | +**登录后:** |
| 123 | + |
| 124 | +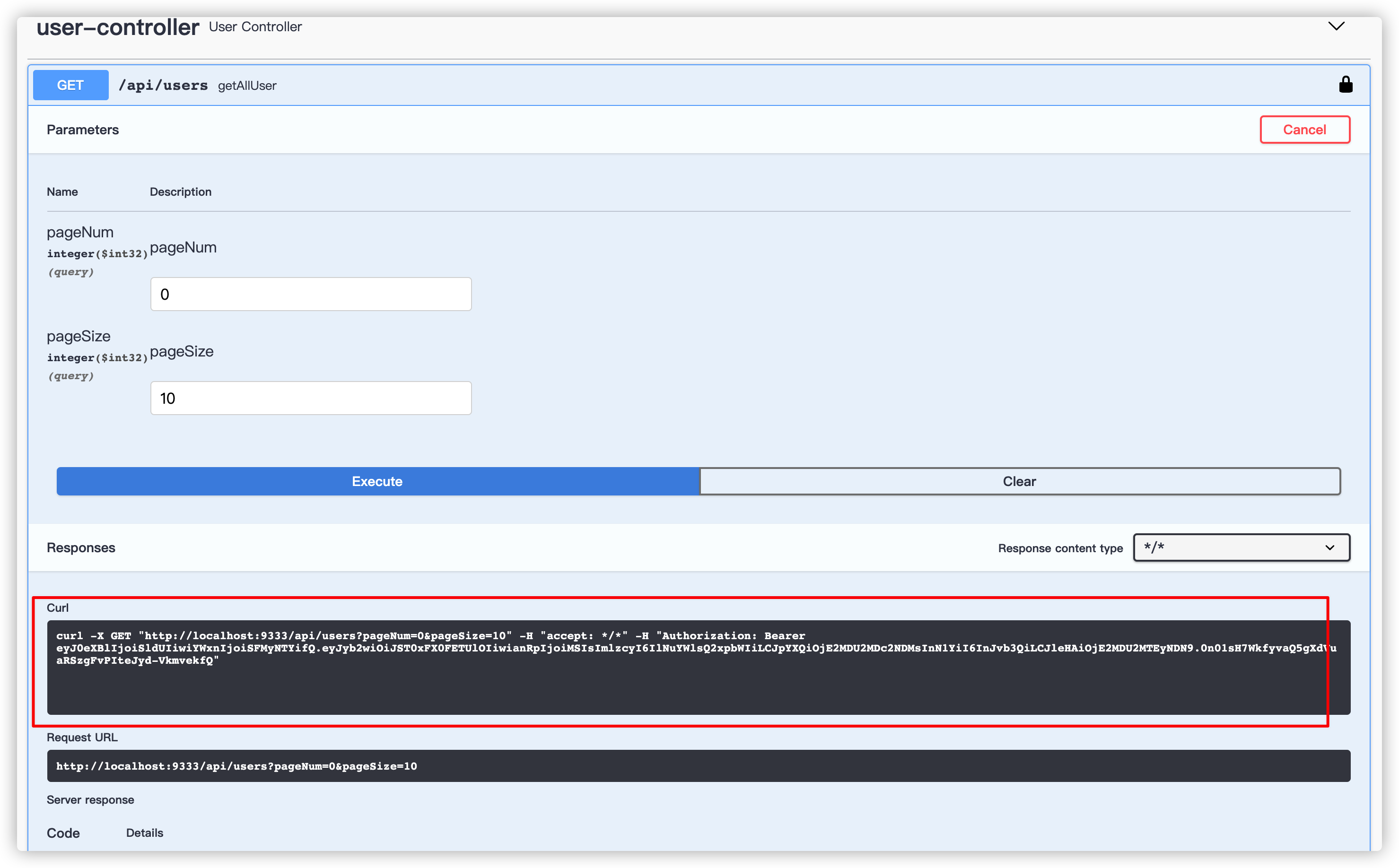 |
| 125 | + |
| 126 | +### 为请求的 Header 添加一个认证参数 |
| 127 | + |
| 128 | +每次请求的时候,我们需要手动输入 token 到指定位置。 |
| 129 | + |
| 130 | +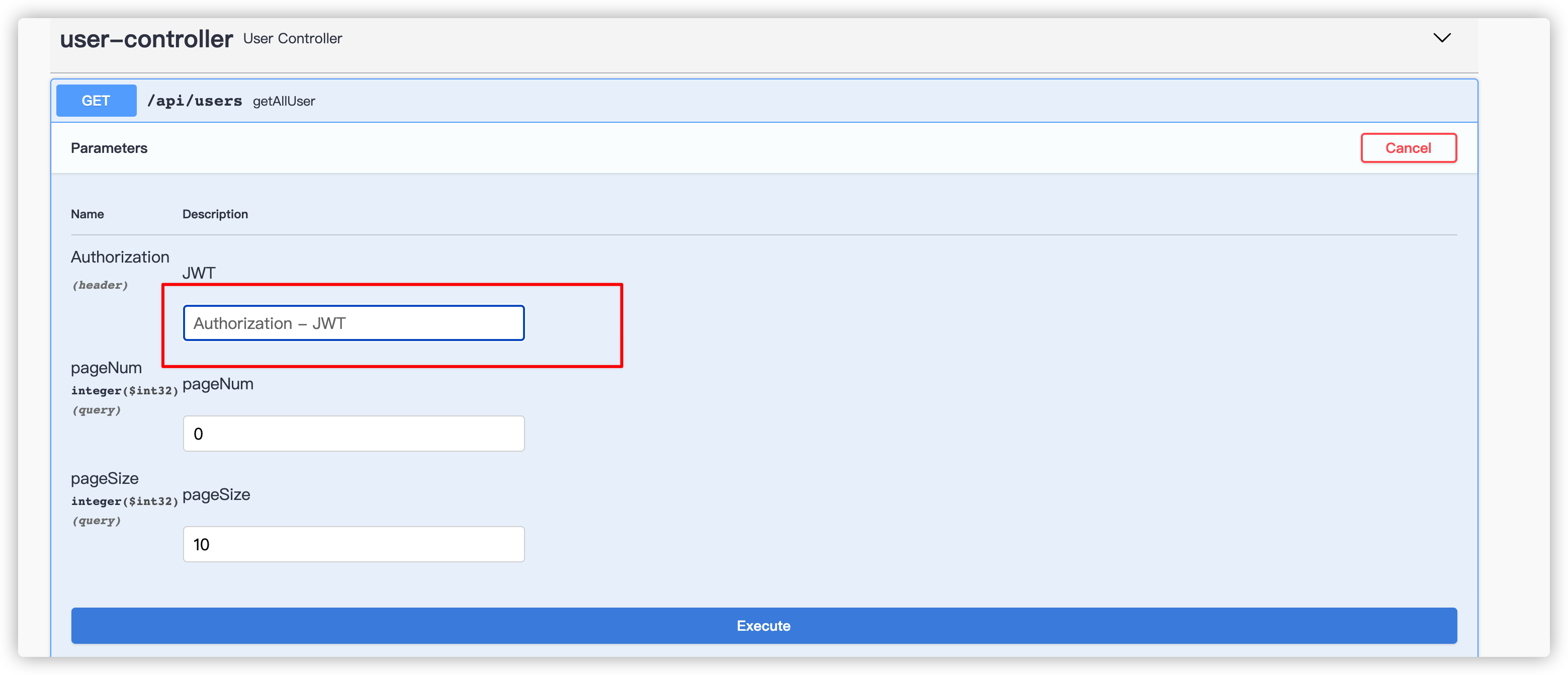 |
| 131 | + |
| 132 | +```java |
| 133 | +@Configuration |
| 134 | +public class SwaggerConfig { |
| 135 | + |
| 136 | + @Bean |
| 137 | + public Docket createRestApi() { |
| 138 | + return new Docket(DocumentationType.SWAGGER_2) |
| 139 | + .apiInfo(apiInfo()) |
| 140 | + .select() |
| 141 | + .apis(RequestHandlerSelectors.basePackage("github.javaguide.springsecurityjwtguide")) |
| 142 | + .paths(PathSelectors.any()) |
| 143 | + .build() |
| 144 | + .globalRequestParameters(authorizationParameter()) |
| 145 | + .securitySchemes(securitySchemes()); |
| 146 | + } |
| 147 | + |
| 148 | + private List<SecurityScheme> securitySchemes() { |
| 149 | + return Collections.singletonList(new ApiKey("JWT", SecurityConstants.TOKEN_HEADER, "header")); |
| 150 | + } |
| 151 | + |
| 152 | + private List<RequestParameter> authorizationParameter() { |
| 153 | + RequestParameterBuilder tokenBuilder = new RequestParameterBuilder(); |
| 154 | + tokenBuilder |
| 155 | + .name("Authorization") |
| 156 | + .description("JWT") |
| 157 | + .required(false) |
| 158 | + .in("header") |
| 159 | + .accepts(Collections.singleton(MediaType.APPLICATION_JSON)) |
| 160 | + .build(); |
| 161 | + return Collections.singletonList(tokenBuilder.build()); |
| 162 | + } |
| 163 | + |
| 164 | + private ApiInfo apiInfo() { |
| 165 | + return new ApiInfoBuilder() |
| 166 | + .title("Spring Security JWT Guide") |
| 167 | + .build(); |
| 168 | + } |
| 169 | +} |
| 170 | +``` |
| 171 | + |
| 172 | +## 使用 knife4j 增强 Swagger |
| 173 | + |
| 174 | +根据官网介绍,knife4j 是为 Java MVC 框架集成 Swagger 生成 Api 文档的增强解决方案。 |
| 175 | + |
| 176 | +项目地址:[https://gitee.com/xiaoym/knife4j](https://gitee.com/xiaoym/knife4j) 。 |
| 177 | + |
| 178 | +使用方式非常简单,添加到相关依赖即可(SpringBoot 版本 2.3.6.RELEASE)。 |
| 179 | + |
| 180 | +```xml |
| 181 | +<dependency> |
| 182 | + <groupId>com.github.xiaoymin</groupId> |
| 183 | + <artifactId>knife4j-spring-boot-starter</artifactId> |
| 184 | + <version>3.0.2</version> |
| 185 | +</dependency> |
| 186 | +``` |
| 187 | + |
| 188 | +完成之后,访问:[http://ip:port/doc.html](http://ip:port/doc.html) 即可。 |
| 189 | + |
| 190 | +效果如下。可以看出,相比于 swagger 原生 ui 确实好看实用了很多。 |
| 191 | + |
| 192 | +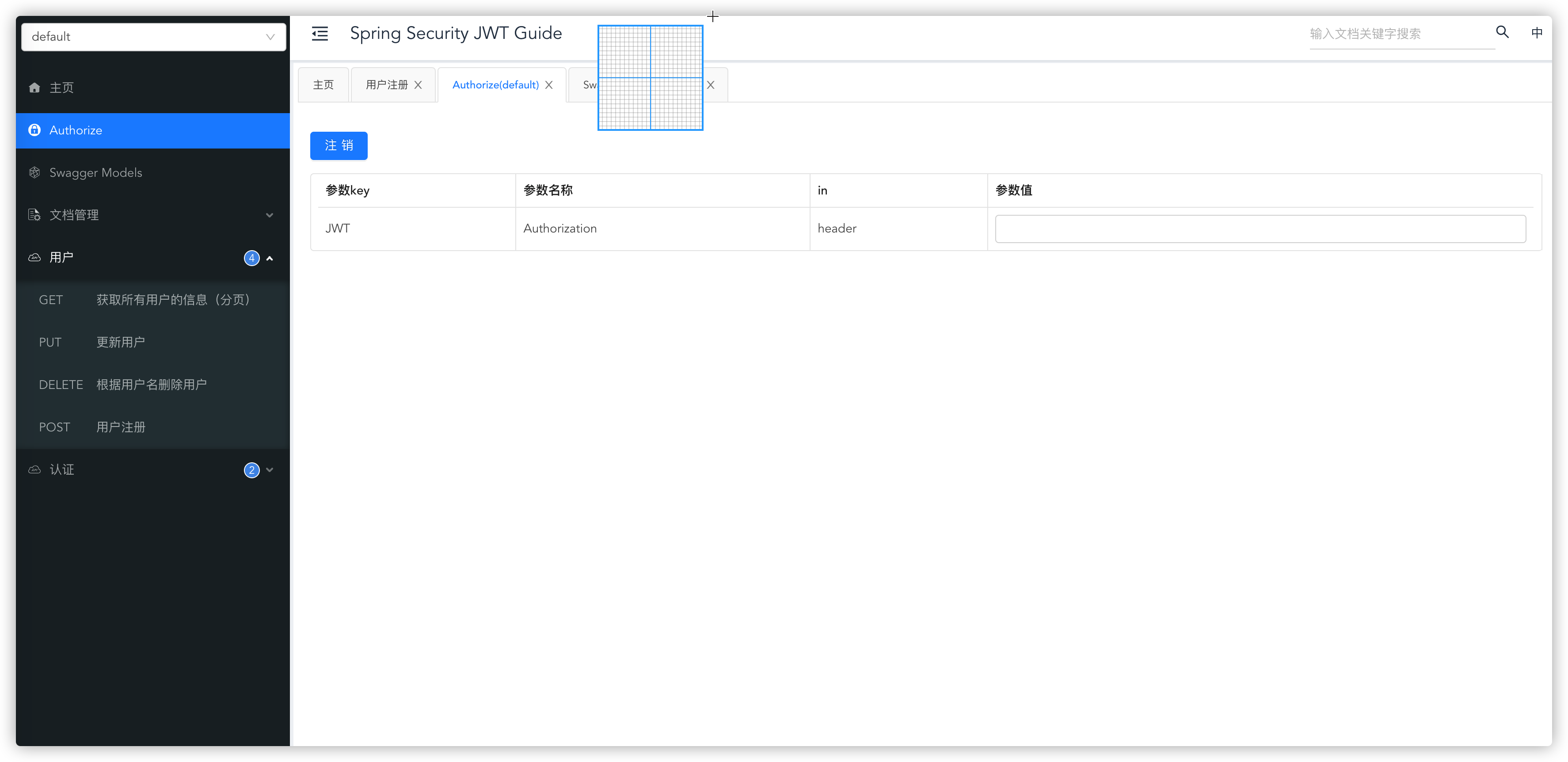 |
| 193 | + |
| 194 | +除了 UI 上的增强之外,knife4j 还提供了一些开箱即用的功能。 |
| 195 | + |
| 196 | +比如:**搜索 API 接口** (`knife4j` 版本>2.0.1 ) |
| 197 | + |
| 198 | +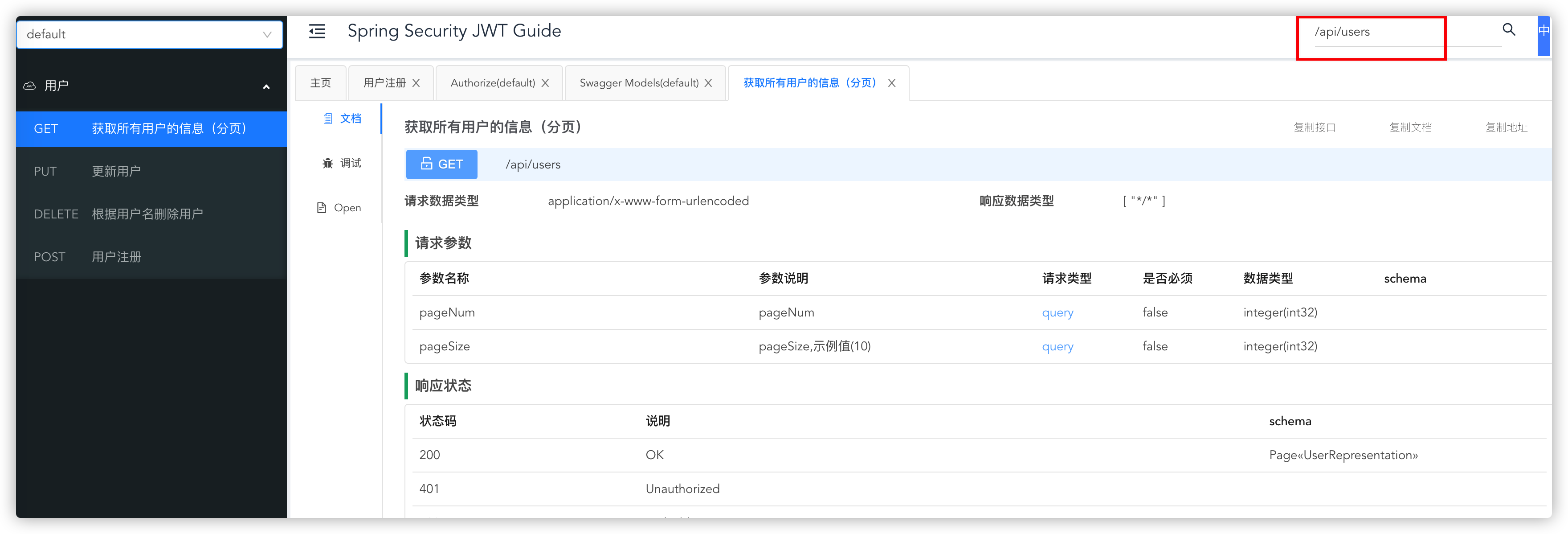 |
| 199 | + |
| 200 | +再比如:**导出离线文档** |
| 201 | + |
| 202 | +通过 `Knife4j` 我们可以非常方便地导出 Swagger 文档 ,并且支持多种格式。 |
| 203 | + |
| 204 | +> - markdown:导出当前逻辑分组下所有接口的 Markdown 格式的文档 |
| 205 | +> - Html:导出当前逻辑分组下所有接口的 Html 格式的文档 |
| 206 | +> - Word:导出当前逻辑分组下所有接口的 Word 格式的文档(自 2.0.5 版本开始) |
| 207 | +> - OpenAPI:导出当前逻辑分组下的原始 OpenAPI 的规范 json 结构(自 2.0.6 版本开始) |
| 208 | +> - PDF:未实现 |
| 209 | +
|
| 210 | +以 HTML 格式导出的效果图如下。 |
| 211 | + |
| 212 | +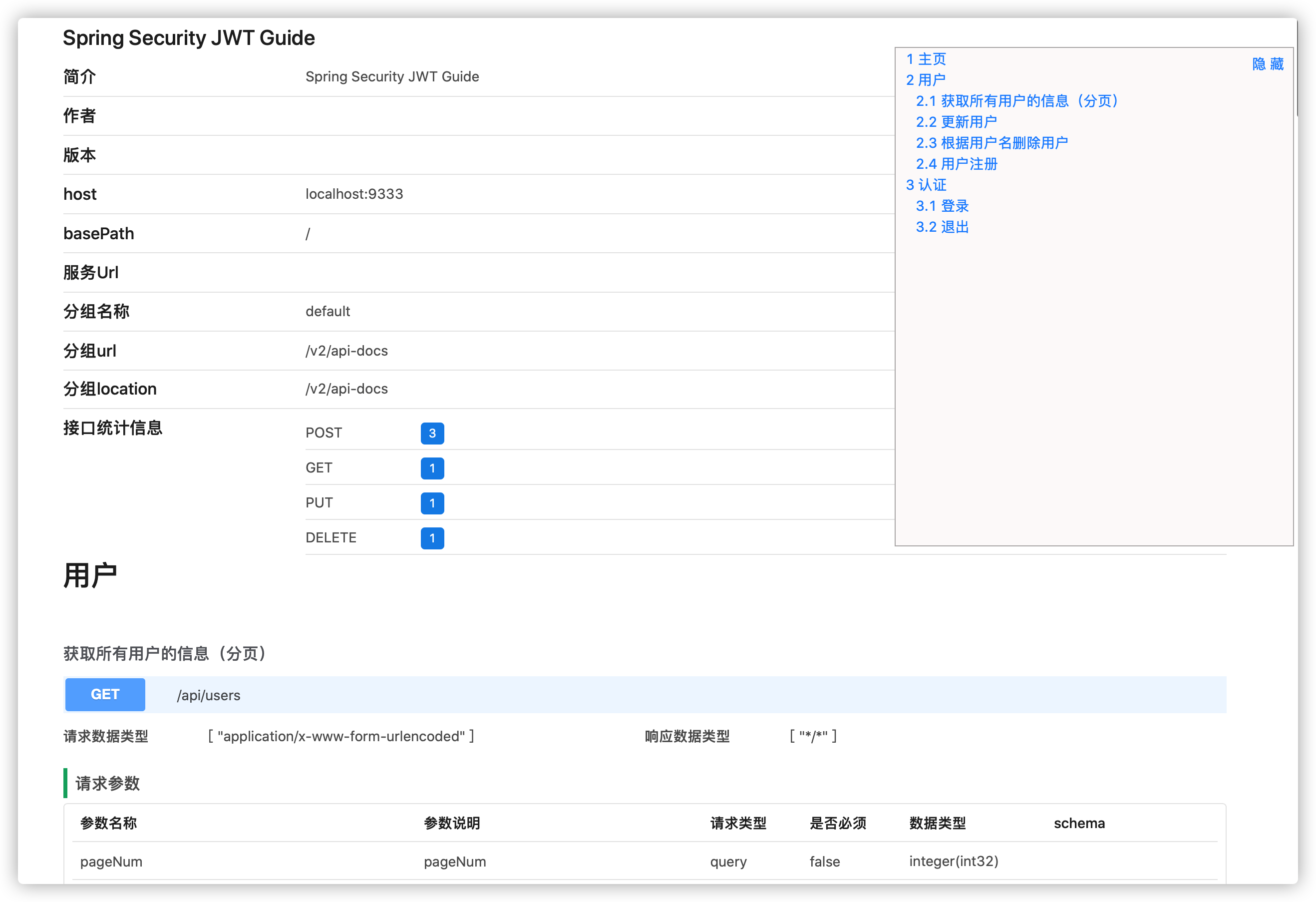 |
| 213 | + |
| 214 | +还等什么?快去试试吧! |
0 commit comments