forked from iree-org/iree
-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Adding HAL profiling API and RenderDoc support for Vulkan. (iree-org#…
…10893) TLDR: configure with `-DIREE_ENABLE_RENDERDOC_PROFILING=ON`, pass the `--device_profiling_mode=queue` flag to the IREE tools, and launch the tools from the RenderDoc UI in order to get a capture (or use `renderdoccmd capture`): 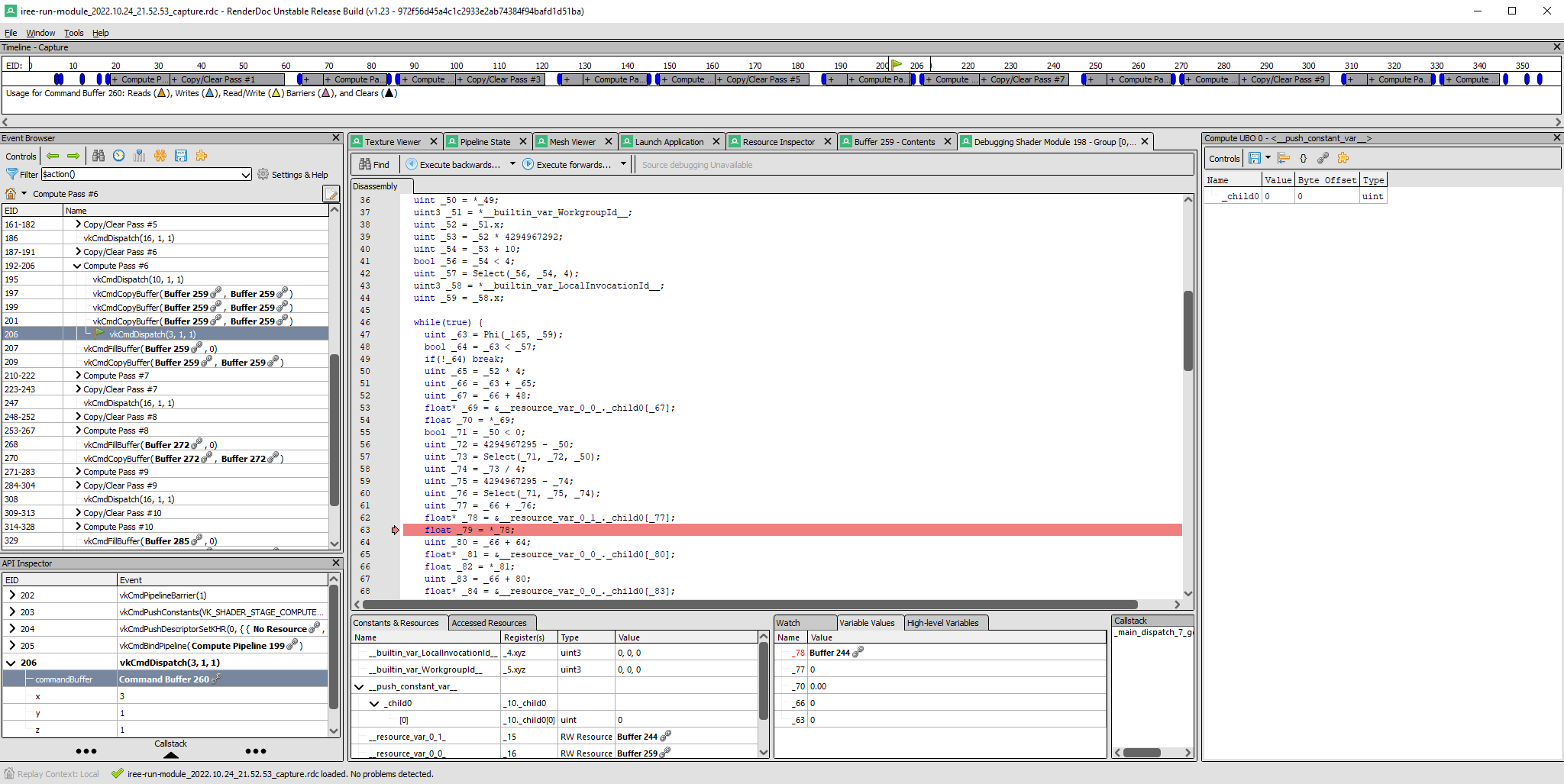 Things are set up to allow for other profiling modes in the future but how best to integrate those is TBD. We can figure out how to scale this with other tooling and on other backends but the rough shape of the API should be compatible with the various backend APIs we target (D3D/Metal/CUDA/Vulkan/perf/etc). Note that because RenderDoc will also capture D3D the cmake flag is generic but both the Vulkan and D3D HAL implementations will need to load it themselves (no real code worth sharing as D3D naturally only needs the Windows API query path). Docs have notes that I've verified on Windows. Someone looking to use this on Android will need to figure that out and can add what they find. Fixes iree-org#45. Forty five. Wow.
- Loading branch information
Showing
21 changed files
with
1,157 additions
and
3 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Oops, something went wrong.