-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Merge pull request ynqa#41 from ynqa/repl
Add REPL
- Loading branch information
Showing
16 changed files
with
477 additions
and
57 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Some generated files are not rendered by default. Learn more about how customized files appear on GitHub.
Oops, something went wrong.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,68 @@ | ||
// Copyright © 2019 Makoto Ito | ||
// | ||
// Licensed under the Apache License, Version 2.0 (the "License"); | ||
// you may not use this file except in compliance with the License. | ||
// You may obtain a copy of the License at | ||
// | ||
// http://www.apache.org/licenses/LICENSE-2.0 | ||
// | ||
// Unless required by applicable law or agreed to in writing, software | ||
// distributed under the License is distributed on an "AS IS" BASIS, | ||
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. | ||
// See the License for the specific language governing permissions and | ||
// limitations under the License. | ||
|
||
package cmd | ||
|
||
import ( | ||
"os" | ||
|
||
"github.com/spf13/cobra" | ||
"github.com/spf13/viper" | ||
|
||
"github.com/ynqa/wego/config" | ||
"github.com/ynqa/wego/repl" | ||
) | ||
|
||
var ReplCmd = &cobra.Command{ | ||
Use: "repl", | ||
Short: "Search similar words with REPL mode", | ||
Long: "Search similar words with REPL mode", | ||
Example: " wego repl -i example/word_vectors.txt\n" + | ||
" >> apple + banana\n" + | ||
" ...", | ||
PreRun: func(cmd *cobra.Command, args []string) { | ||
replBind(cmd) | ||
}, | ||
RunE: func(cmd *cobra.Command, args []string) error { | ||
return executeRepl() | ||
}, | ||
} | ||
|
||
func init() { | ||
ReplCmd.Flags().StringP(config.InputFile.String(), "i", config.DefaultOutputFile, | ||
"input file path for trained word vector") | ||
ReplCmd.Flags().IntP(config.Rank.String(), "r", config.DefaultRank, | ||
"how many the most similar words will be displayed") | ||
} | ||
|
||
func replBind(cmd *cobra.Command) { | ||
viper.BindPFlag(config.InputFile.String(), cmd.Flags().Lookup(config.InputFile.String())) | ||
viper.BindPFlag(config.Rank.String(), cmd.Flags().Lookup(config.Rank.String())) | ||
} | ||
|
||
func executeRepl() error { | ||
inputFile := viper.GetString(config.InputFile.String()) | ||
f, err := os.Open(inputFile) | ||
if err != nil { | ||
return err | ||
} | ||
defer f.Close() | ||
|
||
k := viper.GetInt(config.Rank.String()) | ||
repl, err := repl.NewRepl(f, k) | ||
if err != nil { | ||
return err | ||
} | ||
return repl.Run() | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,34 @@ | ||
// Copyright © 2019 Makoto Ito | ||
// | ||
// Licensed under the Apache License, Version 2.0 (the "License"); | ||
// you may not use this file except in compliance with the License. | ||
// You may obtain a copy of the License at | ||
// | ||
// http://www.apache.org/licenses/LICENSE-2.0 | ||
// | ||
// Unless required by applicable law or agreed to in writing, software | ||
// distributed under the License is distributed on an "AS IS" BASIS, | ||
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. | ||
// See the License for the specific language governing permissions and | ||
// limitations under the License. | ||
|
||
package cmd | ||
|
||
import ( | ||
"testing" | ||
|
||
"github.com/spf13/viper" | ||
) | ||
|
||
const replFlagSize = 2 | ||
|
||
func TestReplBind(t *testing.T) { | ||
defer viper.Reset() | ||
|
||
replBind(ReplCmd) | ||
|
||
if len(viper.AllKeys()) != replFlagSize { | ||
t.Errorf("Expected replBind maps %v keys: %v", | ||
replFlagSize, viper.AllKeys()) | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,45 @@ | ||
# REPL | ||
|
||
REPL mode for similarity search | ||
|
||
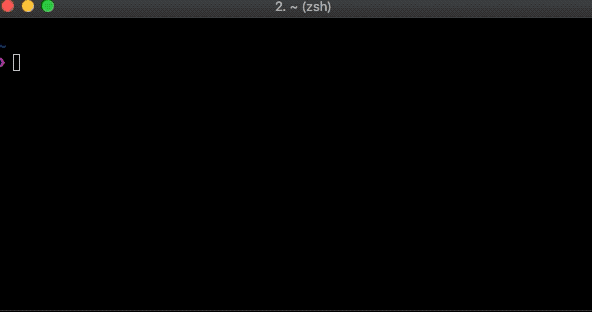 | ||
|
||
## Usage | ||
|
||
``` | ||
Search similar words with REPL mode | ||
Usage: | ||
wego repl [flags] | ||
Examples: | ||
wego repl -i example/word_vectors.txt | ||
>> apple + banana | ||
... | ||
Flags: | ||
-h, --help help for repl | ||
-i, --inputFile string input file path for trained word vector (default "example/word_vectors.txt") | ||
-r, --rank int how many the most similar words will be displayed (default 10) | ||
``` | ||
|
||
## Example | ||
|
||
Now, it is able to use `+`, `-` for arithmetic operations. | ||
|
||
``` | ||
$ go run wego.go repl -i example/word_vectors_sg.txt | ||
>> a + b | ||
RANK | WORD | SIMILARITY | ||
+------+---------+------------+ | ||
1 | phi | 0.907975 | ||
2 | q | 0.904593 | ||
3 | mathbf | 0.903066 | ||
4 | cdot | 0.902205 | ||
5 | b | 0.901952 | ||
6 | becomes | 0.900346 | ||
7 | int | 0.898680 | ||
8 | z | 0.897895 | ||
9 | named | 0.896480 | ||
10 | v | 0.895456 | ||
``` |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,40 @@ | ||
// Copyright © 2019 Makoto Ito | ||
// | ||
// Licensed under the Apache License, Version 2.0 (the "License"); | ||
// you may not use this file except in compliance with the License. | ||
// You may obtain a copy of the License at | ||
// | ||
// http://www.apache.org/licenses/LICENSE-2.0 | ||
// | ||
// Unless required by applicable law or agreed to in writing, software | ||
// distributed under the License is distributed on an "AS IS" BASIS, | ||
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. | ||
// See the License for the specific language governing permissions and | ||
// limitations under the License. | ||
|
||
package repl | ||
|
||
type Operator func(float64, float64) float64 | ||
|
||
func elementWise(v1, v2 []float64, op Operator) []float64 { | ||
for i := 0; i < len(v1); i++ { | ||
v1[i] = op(v1[i], v2[i]) | ||
} | ||
return v1 | ||
} | ||
|
||
func addOp(x, y float64) float64 { | ||
return x + y | ||
} | ||
|
||
func Add(v1, v2 []float64) []float64 { | ||
return elementWise(v1, v2, addOp) | ||
} | ||
|
||
func subOp(x, y float64) float64 { | ||
return x - y | ||
} | ||
|
||
func Sub(v1, v2 []float64) []float64 { | ||
return elementWise(v1, v2, subOp) | ||
} |
Oops, something went wrong.