forked from async-rs/async-std
-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
125: from/into stream r=yoshuawuyts a=yoshuawuyts This adds `Stream` counterparts to `FromIterator`, `IntoIterator` and `Iterator::collect`, allowing to use the same patterns that are common in streams. Thanks! ## Tasks - [x] `FromStream` - [x] `IntoStream` - [x] `Stream::collect` ## Screenshot 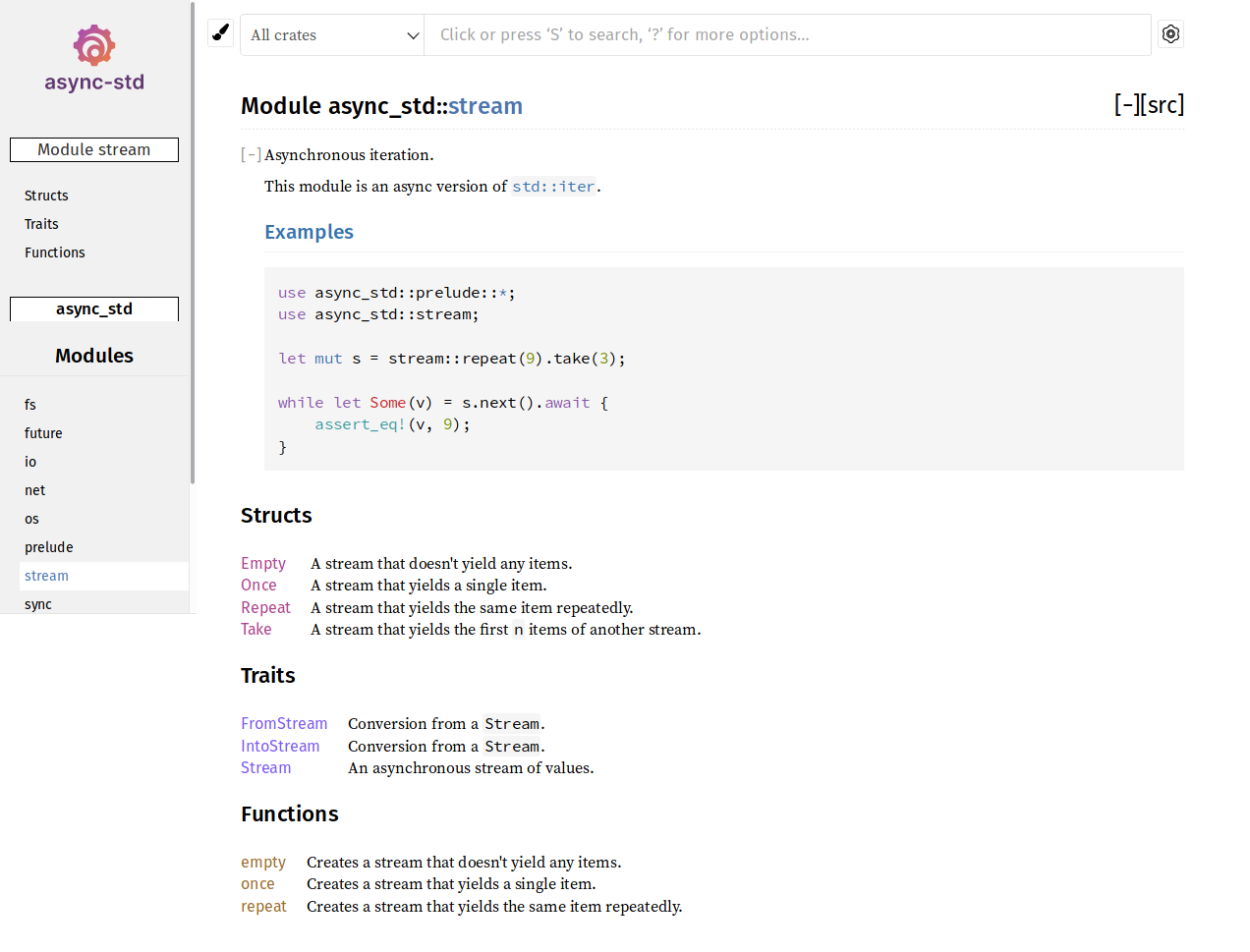 Co-authored-by: Yoshua Wuyts <[email protected]>
- Loading branch information
Showing
7 changed files
with
164 additions
and
3 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,29 @@ | ||
use super::IntoStream; | ||
|
||
use std::pin::Pin; | ||
|
||
/// Conversion from a `Stream`. | ||
/// | ||
/// By implementing `FromStream` for a type, you define how it will be created from a stream. | ||
/// This is common for types which describe a collection of some kind. | ||
/// | ||
/// See also: [`IntoStream`]. | ||
/// | ||
/// [`IntoStream`]: trait.IntoStream.html | ||
#[cfg_attr(feature = "docs", doc(cfg(unstable)))] | ||
pub trait FromStream<T: Send> { | ||
/// Creates a value from a stream. | ||
/// | ||
/// # Examples | ||
/// | ||
/// Basic usage: | ||
/// | ||
/// ``` | ||
/// // use async_std::stream::FromStream; | ||
/// | ||
/// // let _five_fives = async_std::stream::repeat(5).take(5); | ||
/// ``` | ||
fn from_stream<'a, S: IntoStream<Item = T> + Send + 'a>( | ||
stream: S, | ||
) -> Pin<Box<dyn core::future::Future<Output = Self> + Send + 'a>>; | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,36 @@ | ||
use futures_core::stream::Stream; | ||
|
||
/// Conversion into a `Stream`. | ||
/// | ||
/// By implementing `IntoIterator` for a type, you define how it will be | ||
/// converted to an iterator. This is common for types which describe a | ||
/// collection of some kind. | ||
/// | ||
/// [`from_stream`]: #tymethod.from_stream | ||
/// [`Stream`]: trait.Stream.html | ||
/// [`collect`]: trait.Stream.html#method.collect | ||
/// | ||
/// See also: [`FromStream`]. | ||
/// | ||
/// [`FromStream`]: trait.FromStream.html | ||
#[cfg_attr(feature = "docs", doc(cfg(unstable)))] | ||
pub trait IntoStream { | ||
/// The type of the elements being iterated over. | ||
type Item; | ||
|
||
/// Which kind of stream are we turning this into? | ||
type IntoStream: Stream<Item = Self::Item> + Send; | ||
|
||
/// Creates a stream from a value. | ||
fn into_stream(self) -> Self::IntoStream; | ||
} | ||
|
||
impl<I: Stream + Send> IntoStream for I { | ||
type Item = I::Item; | ||
type IntoStream = I; | ||
|
||
#[inline] | ||
fn into_stream(self) -> I { | ||
self | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,25 @@ | ||
use crate::stream::{FromStream, IntoStream, Stream}; | ||
|
||
use std::pin::Pin; | ||
|
||
impl<T: Send> FromStream<T> for Vec<T> { | ||
#[inline] | ||
fn from_stream<'a, S: IntoStream<Item = T>>( | ||
stream: S, | ||
) -> Pin<Box<dyn core::future::Future<Output = Self> + Send + 'a>> | ||
where | ||
<S as IntoStream>::IntoStream: Send + 'a, | ||
{ | ||
let stream = stream.into_stream(); | ||
|
||
Pin::from(Box::new(async move { | ||
pin_utils::pin_mut!(stream); | ||
|
||
let mut out = vec![]; | ||
while let Some(item) = stream.next().await { | ||
out.push(item); | ||
} | ||
out | ||
})) | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,9 @@ | ||
//! The Rust core allocation and collections library | ||
//! | ||
//! This library provides smart pointers and collections for managing | ||
//! heap-allocated values. | ||
mod from_stream; | ||
|
||
#[doc(inline)] | ||
pub use std::vec::Vec; |